Golang多线程下载文件
在现代的网络环境中,我们经常需要下载大文件。传统的单线程下载方式往往效率低下,因为它们无法充分利用计算机的多核处理能力。而Golang作为一门支持多线程编程的语言,可以帮助我们实现高效的多线程下载。本文将介绍如何使用Golang进行多线程下载文件。
使用goroutine并发下载
在Golang中,我们可以使用goroutine来实现并发请求。通过创建多个goroutine并行下载文件的不同部分,我们可以利用计算机的多核心处理器,提高文件下载速度。
首先,我们需要确定要下载的文件的URL和文件大小。然后,我们可以使用http包来发送HTTP请求并获取文件的内容。
为了实现多线程下载,我们可以将文件拆分成多个部分,并为每个部分创建一个goroutine来下载。例如,如果文件大小是1GB,我们可以将其分成10个100MB的部分,为每个部分创建一个goroutine。
以下是一个简单的例子,演示如何使用goroutine并发下载文件:
package main
import (
"fmt"
"io/ioutil"
"net/http"
"os"
"sync"
)
func main() {
fileURL := "http://example.com/large-file.zip"
fileSize := 1024 * 1024 * 1024 // 1GB
numOfThreads := 10
threadSize := fileSize / numOfThreads
var wg sync.WaitGroup
wg.Add(numOfThreads)
for i := 0; i < numofthreads;="" i++="" {="" go="" func(startindex="" int)="" {="" defer="" wg.done()="" endindex="" :="startIndex" +="" threadsize="" -="" 1="" create="" a="" new="" http="" request="" req,="" err="" :="http.NewRequest("GET"," fileurl,="" nil)="" if="" err="" !="nil" {="" fmt.println("failed="" to="" create="" http="" request:",="" err)="" return="" }="" specify="" the="" range="" of="" bytes="" to="" download="" rangeheader="" :="fmt.Sprintf("bytes=%d-%d"," startindex,="" endindex)="" req.header.set("range",="" rangeheader)="" send="" the="" http="" request="" client="" :="&http.Client{}" resp,="" err="" :="client.Do(req)" if="" err="" !="nil" {="" fmt.println("failed="" to="" send="" http="" request:",="" err)="" return="" }="" defer="" resp.body.close()="" read="" the="" response="" body="" body,="" err="" :="ioutil.ReadAll(resp.Body)" if="" err="" !="nil" {="" fmt.println("failed="" to="" read="" response="" body:",="" err)="" return="" }="" save="" the="" downloaded="" part="" to="" a="" file="" outputfile,="" err="" :="os.OpenFile(fmt.Sprintf("part%d.bin"," i),="" os.o_create|os.o_wronly,="" 0666)="" if="" err="" !="nil" {="" fmt.println("failed="" to="" open="" output="" file:",="" err)="" return="" }="" defer="" outputfile.close()="" write="" the="" downloaded="" part="" to="" the="" output="" file="" _,="" err="outputFile.Write(body)" if="" err="" !="nil" {="" fmt.println("failed="" to="" write="" to="" output="" file:",="" err)="" return="" }="" fmt.println("downloaded="" part",="" i)="" }(i="" *="" threadsize)="" }="" wg.wait()="" fmt.println("download="" completed")="" }="">
通过运行以上程序,我们可以使用10个goroutine并发地下载一个1GB的文件。每个goroutine将负责下载一个100MB的部分。当所有goroutine都完成下载后,我们将得到完整的文件。
总结
Golang的goroutine提供了一种方便且高效的方式来实现多线程编程。通过将文件分成多个部分,并为每个部分创建一个goroutine来下载,我们可以利用计算机的多核处理能力,提高文件下载的速度。希望本文对你理解如何使用Golang进行多线程下载有所帮助。
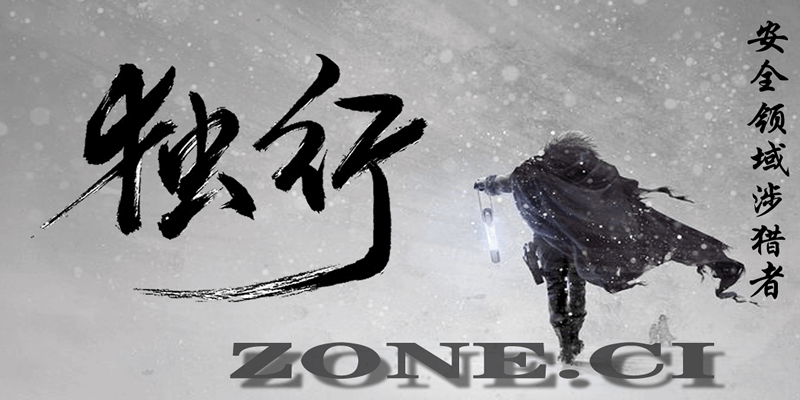
版权声明
本站原创文章转载请注明文章出处及链接,谢谢合作!
评论