Introduction
Golang (or Go) is a powerful programming language that has gained significant popularity in recent years. It is known for its simplicity, speed, and robustness. One area where it shines is network programming, specifically HTTP services. In this article, we will explore how to handle HTTP chunked transfer encoding in Golang using the built-in "net/http" package.
Understanding HTTP Chunked Transfer Encoding
HTTP Chunked Transfer Encoding is a mechanism used to send a large payload in smaller "chunks" over an HTTP connection. This can be useful when the size of the payload is unknown or when the server wants to start sending the response before generating the entire payload. The server breaks the payload into smaller chunks and sends them one by one, with each chunk preceded by its size in hexadecimal format.
Implementing HTTP Chunked Transfer Encoding in Golang
In Golang, handling HTTP Chunked Transfer Encoding is straightforward thanks to the "net/http" package. Let's explore how we can implement it:
Sending Chunked Responses
To send chunked responses from the server, we need to set the "Transfer-Encoding" header to "chunked" and write the chunks. Here's a basic example:
```go func handler(w http.ResponseWriter, r *http.Request) { w.Header().Set("Transfer-Encoding", "chunked") // Write the chunks fmt.Fprint(w, "6\r\nChunk 1\r\n") time.Sleep(time.Second) // Simulating delay between chunks fmt.Fprint(w, "7\r\nChunk 2\r\n") time.Sleep(time.Second) // Simulating delay between chunks fmt.Fprint(w, "0\r\n\r\n") // Final chunk with size of 0 indicates end of chunks } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } ```Receiving Chunked Responses
When receiving a chunked response in Golang, the "Transfer-Encoding" header is automatically handled by the "net/http" package. We can read the chunks using the "io" package's "Read()" function. Here's an example:
```go func main() { resp, err := http.Get("http://example.com") if err != nil { log.Fatal(err) } defer resp.Body.Close() // Read chunks from the response body var buf bytes.Buffer chunk := make([]byte, 256) for { n, err := resp.Body.Read(chunk) if n > 0 { buf.Write(chunk[:n]) fmt.Printf("Received chunk: %s\n", chunk[:n]) } if err == io.EOF { break } if err != nil && err != io.EOF { log.Fatal(err) } } fmt.Printf("Final response: %s\n", buf.String()) } ```Conclusion
HTTP Chunked Transfer Encoding is a useful technique for handling large payloads over HTTP connections. In this article, we learned how to implement HTTP Chunked Transfer Encoding in Golang using the built-in "net/http" package. We explored how to send and receive chunked responses. Golang's simplicity and powerful standard library make it an excellent choice for developing robust and efficient HTTP services.
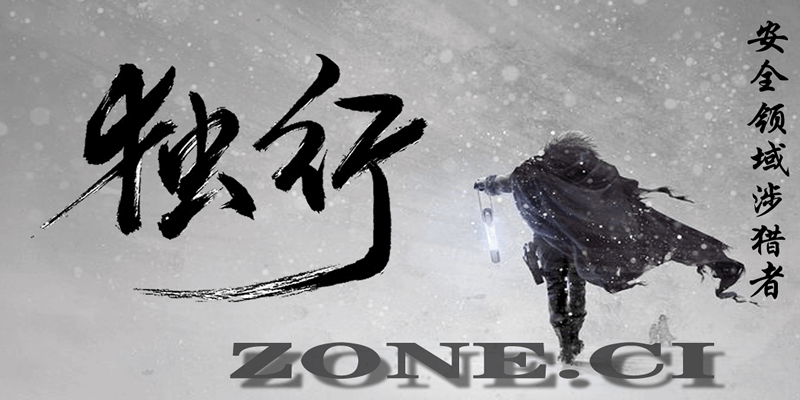
评论